The PHP File_Get_Contents() Function - Read File Into A String - PHP Tutorials
Using the File_Get_Contents() function to read file contents into a string.
Let’s take a look at how we can use the php file_get_contents function as the preferred way to read an entire file's contents into a PHP string.
PHP Str_Replace Function Introduction & Syntax
The PHP file_get_contents() function is a built-in PHP function that allows you to read a file's content into a string variable. This method is the preferred way to read a file into a string as it provides support to memory mapping techniques for specific operating systems to enhance performance.

Parameters For The File_Get_Contents() Function:
The first parameter known as $filename is mandatory with the other 4 being optional.
FileName
This is the name of the file you want to read. This parameter can also be a directory to read from following standard PHP directory reading requirements.
Use_Include_Path
This is an optional parameter that can be used to also search for the given $filename in the include path. You cannot use the PHP constant FILE_USE_INCLUDE_PATH if strict typing is enabled when calling this function given the constant is an int. Instead set $use_include_path to True.
Context
This optional parameter allows you to pass a valid context resource created with stream_context_create(). If this is not needed, set this equal to Null.
Offset
An optional parameter that can be used to set where reading the original stream starts. Providing a negative value for offset counts from the end of the stream. Support for this optional parameter as a negative value was added in the version 7.1.0 PHP update.
Length
Length parameter allows you to set a maximum amount of data to read from the contents of the file. This parameter defaults to read the entire file length to the end. This parameter was added in the version 5.1 PHP update.
Return Values For File_Get_Contents() Function:
The file_gets_contents function returns the read in data or a boolean false if it failed.
Quick Notes About The PHP File_Get_Contents() Function:
Seeking for the specified offset parameter is not fully supported with remote files such as S3 on AWS. This process might work on remote files using smaller offset values, but can be difficult to predict with the use of buffered streams.
The length parameter is applied to the file reading stream processed by the filters.
If you’re looking to use a file pointer instead of a path or url use the fgets() function instead.
The file_get_contents() function by default returns false when the function failed to read the file. With that in mind there are situations where it may return a non-boolean value that evaluates to false. The language type guide provided by the PHP site provides more information on boolean values. It’s recommended that you use the ‘===’ operation when evaluating the return value of the file_get_contents() function.
You can only use a website URL as the filename for the file_get_contents() function if fopen() wrappers have been enabled. Examples of supported fopen() wrappers include:
- file://
- http://
- ftp://
- php://

This function is also considered to be binary-safe.
Commonly Seen Errors and Exceptions:
It’s highly recommended you encode your file with urlencode() when opening a file with special characters.
The function will return an E_WARNING level error if the function cannot find the file, the length to read in is less than 0, or if the specific offset cannot be located in the stream and fails.
The commonly seen warning “file_get_contents(www.google.com) [function.file-get-contents]: failed to open stream” can occur when removing wrappers such as ‘http://’ from the given $filename url. This warning can be suppressed by adding an error control operator in front of the function call where the $filename is passed.
PHP File_Get_Contents() Function Examples:
Let’s look at a wide variety of ways to use the php file_get_contents() function to read data into a string.
Simple PHP File_Get_Contents() Examples:
This code example reads the contents of a text file into a string variable $result. Only parameter used is the mandatory $filename location.
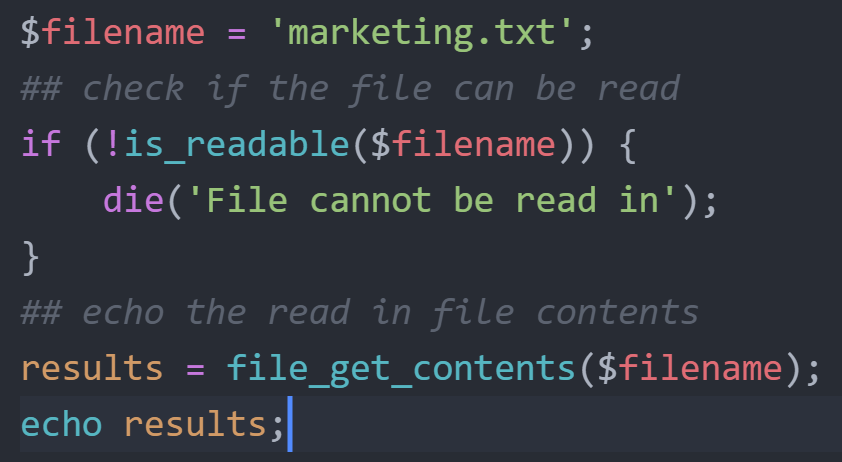
You can use the is_readable() function as a check before running the file_get_contents() to ensure access to the file contents. Is_readable() will check if the file exists and if it has readable properties.
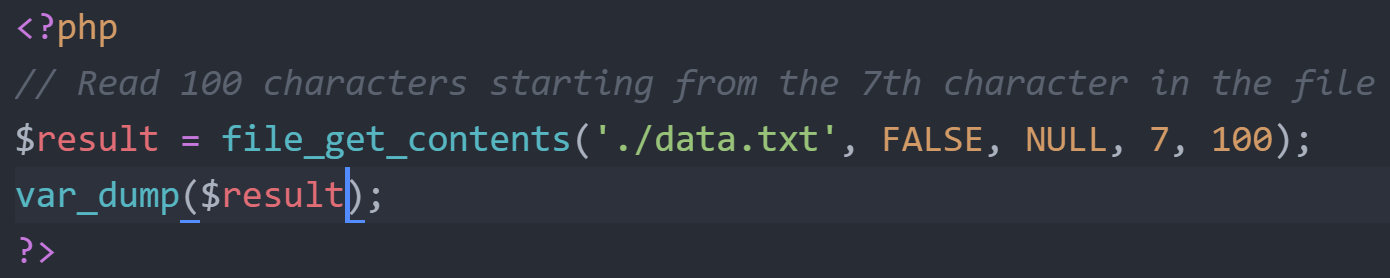
The above example allows us to read a specific section of the data.txt file starting at the 7th character in the file through the next 100. Remember this function can fail if the file contains less than 100 characters.
Using File_Get_Contents() With A HTTPS Url To Read Website Data
This example below uses the file_get_contents() function to read an entire https:// url for a webpage into a string variable.

With the file_get_contents() function and an https:// url we can create a simple POST request. We have to create a custom $context to pass our request array into the file_get_contents and can do that with the stream_context_create() php function. Given the situation and context of what you’re reading into the string result you can use http:// or php:// as well. The function will return an error message if the stream fails to be created.

Quick note worth mentioning: You can pass authentication information such as username and password in the url with HTTP basic access authentication, but cannot do this for HTTP “Digest” access authentication. With that said it’s always preferred to pass authentication in the format above.
Receiving A JSON POST With File_Get_Contents(‘php://input’)
The php://input fopen() wrapper is a read-only stream that can read in data from a json POST request body. It will return any raw data after HTTP headers. As you may know you can use the $_POST[] global variable to receive post data, but this does not work when we want to receive a json string.

Our results come back as a string variable in the json format and can use json_decode() to convert this string into a json object. The //filter wrapper allows you to filter the incoming data.
Deeper Examples Of The PHP File_Get_Contents() Function
Let’s look at a few longer examples of using the file_get_contents() function to read an entire file into a string.
File Contents Encoding Check & Conversion With File_Get_Contents()
We can use this function along with mb_detect_encoding() & iconv() to check the encoding type of a file's contents and convert it to UTF-8. This function is used as a part of a CSV reader used for accessing local data.

Note: You might want to set a second parameter to ‘True’ in the json_decode function as this will return a type object by default instead of the expected array. Be careful with what your $searchValue and $replaceValue are as well. If they contain strings that match json encoding it can lead to issues.
Using hoa:// To Read Entire Files Of Database JSON With File_Get_Contents()
This example function allows us to use file_get_contents() and the ‘hoa://’ wrapper to read json data from a database path. After we decode the newly received $dates data we return a json encoded array_slice()

Important Information For Using File_Get_Contents('php //input')
The php://input wrapper allows you to read the raw request body from a JSON request. Two of the key benefits to using php:// is it does not need any special php.ini directives to work and is less memory intensive then other options such as $http_raw_post_data (which has been deprecated). This wrapper is also considered a read-only stream.
When using php://input as a part of a request the content that is trying to be streamed through will not get through if the request is redirected. It’s good to keep this in mind when writing complex requests and not seeing data come through with php://input. This issue can come up when doing a redirect as simple as http:// to https://.
Summary
The File_Get_Contents() function is the best way to read an entire file’s contents into a string and interact with the data in various ways in PHP. This function can be used for tasks as simple as reading a simple text file into a string to building custom context resources to process POST/GET/PUT requests to reach non local files. The