PHP Password_Verify() Function - Verify That A Hash Matches A Given Password
Let's take a look at how we can use the password_verify() function to match a hash and password str
We're going to look at how we can use the password_verify() function to verify that a given password matches a previously hashed password. We'll walk through some examples to see how this function can be used in a production setting.
PHP Password_Verify() Function Introduction & Syntax
The PHP password_verify() function is a built-in function that was introduced with PHP 5.5.0. This function is used to verify a given password against a password hash created by the password_hash() function. A typical workflow with the password_verify function might look something like this: User submits their login credentials to the login page. Their inputted password gets hashed using the password_hash() function. This hash gets stored in our user database. The stored password hash gets compared to the newly inputted password hash created with password_hash(). This comparison is done using the password_verify function.
Parameters For The Password_Verify() Function
The first parameter is the given password that needs to be verified against the second parameter, the hash. Both of these parameters are mandatory.
Password String
The first parameter is the string version of the password we want to compare. This parameter is mandatory.
Hash
The $hash parameter is our password hash created using the password_hash() function which uses the bcrypt hashing algorithm. This parameter is mandatory.
Return Values For The Password_Verify() Function
The function returns either a boolean true or false based on if the $hash could be verified with the given $password. If the $hash has been salted, then the $password must include the same salt at the beginning of the password and may also contain data such as the algorithm, cost, and salt.
Quick Notes About The PHP Password Verify Function
This function checks for a hash match between the two string variables. This function will return false if $hash is of an invalid format. This function uses the timing attack safe string comparison function provided by hash_equals().
PHP Password_Verify() Example
Let’s look at an example of how we can use the password_verify() function to compare a string variable to a hash.
Simple Password_Verify() Comparison Example
This 3 step example below creates a new user’s $password and stores a password string inside of it. Then we create another variable $hash and store the password_hash() function output. This function returns the cryptological hash of our $password variable which we can store and use to verify against user input later.
The result of this code will return a hash of our $password variable. This is useful for storing in a database for comparison later against a user input value.
Verify Submitted Password Against Hash
This example below builds on the previous one and uses the $hash to verify the password against user input. In this case, we’re using the same password but you could use different ones.
The result of this code snippet is ‘Valid password.’ If we were to change our $password variable to ‘notmypassword’ we would see ‘Invalid password.’ displayed.
Upgrade Hash If Hash Needs Rehashing
This final simple code example builds on previous ones and demonstrates how to verify a password and if need be rehash it to upgrade the encryption.
The result of this code is ‘Password is valid!’. This lets us know that our hash is working correctly. If we need to update the hash, we can do so by checking if password_needs_rehash returns true and perform an update
Deeper Examples of Password_Verify() Function
A few more quick use cases that provide real world application.
Verify password against database records
If you are verifying a password against a database, use password_verify(). If you are just verifying two passwords entered by the user (e.g. during registration), use hash_equals().
This is also useful if you need to verify multiple passwords. Just hash each password with password_hash() and compare them with hash_equals().
PHP password_verify() forgot password example
When a user forgets their password, they will enter their email address on the forgot password page. Then, we will look up their account in the database and send them an email with a link to reset their password.
In the code above, we are using PHP’s mail() function to send an email to the user with a link to reset their password. The link will include a random string of characters (which we generated with PHP’s str_shuffle() and substr() functions) and the user’s email address. When the user clicks on the link, they will be taken to a reset.php page, where we will verify the key and email address before allowing the user to change their password.
Summary
The Password_Verify() function is a great way to verify that a given password matches a stored password hash. The function takes the given password and compares it to the password hash created by the password_hash() function. If the two match, then the function returns true, otherwise it returns false.
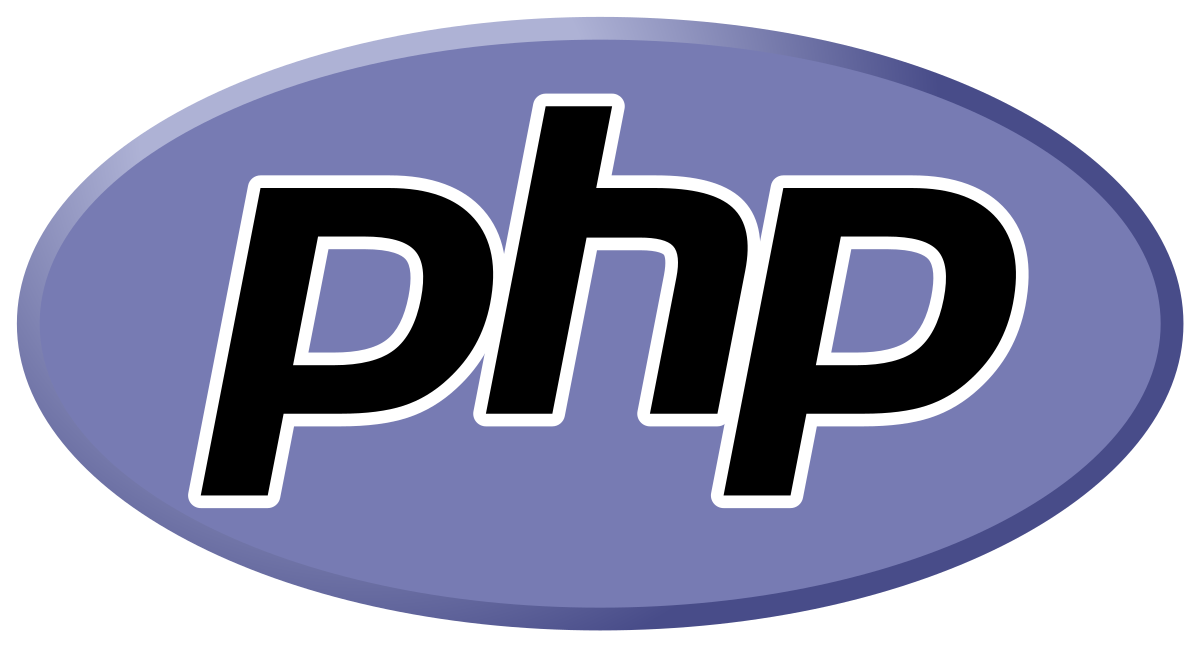