PHP Array_Merge() Function - Merge One or More Arrays Into One Array
Let's take a look at how we can use the php array_merge() function to merge arrays into one array.
We’re going to take a look at how we can use the php array_merge() function to merge one, two, or multiple arrays into one array. We’ll walk through various implementations and examples that show the extension of this php function.
PHP Array_Merge() Function Introduction & Syntax
The PHP Array_Merge() function is a built-in php function that is used to merge the elements of one, two, or multiple arrays together into a single array. The elements of the arrays are appended to the end of the previous array in the input list. The function returns the resulting array, which will be empty if no arrays are added as arguments.
Parameters For Array_Merge() Function:
The only parameters are the given arrays you want to merge together, in the order you want them to concatenate to each other. These arrays are separated by commas.
$Arrays
This is a variable list of arrays to merge together into a single array. If the elements in the arrays have string keys and two lists have the same string keys the later value for that key will overwrite the previous ones. In the case the arrays contain numeric keys the later element value will not overwrite the other values and will just be appended. The values with numeric keys will be renumbered with new incrementing keys starting at 0 in the resulting array.
The result from this array merge is an array with one element:( [0] => data ).
Return Value For Array_Merge() Function:
The function returns a new array made up of concatenated elements from the input arrays. If no arrays are added as inputs the function will return an empty array.
Quick Things To Know About Array_Merge() Function:
PHP version 7.4.0 added the ability to call this function without any parameters.
Array_merge() was added in PHP 4.
While the array_merge() function appends array elements in order, the array_merge_recursive() function merges the arrays recursively.
If only one array is passed the function will just return the same array.
PHP Array_Merge() Function Examples:
Let’s look at a wide variety of ways we can use the array_merge() function to merge multiple arrays into one.
Simple Array_Merge() Function Examples:
This code example merges our two arrays together using two simple arrays.
The result array looks like this:
Notice how the result array adds numeric keys when the passed arrays do not have keys.
Using The Array_Merge() Function With String Keys
This example shows how the array_merge() function removes the duplicate values when the string key is an exact match between the two arrays. The function uses the later value between the two array elements.
The output from the above example:
You’ll notice that for the two elements that have the same key the array_merge() function uses the later value in the arrays, but still puts them in order given that both those string keys appear before anything else in the first array. Once those values are changed the rest of the elements are concatenated in order.
Merge 2 Arrays With Numeric Keys With Array_Merge()
Here’s an example of what happens when we merge 2 arrays with numeric keys together.
The resulting array is:
You’ll notice numeric keys that are the same do not get combined and values replaced like with string keys. The function more or less ignores the numeric keys and just concatenates the two arrays values in order with new keys starting from zero.
Tips From A Real Data Processing Use Case
One of the workflow processes used in systems like this product recognition software build is managing multiple extending pipelines all related to the same input, usually based on a unique_id(). Input data such as an image would come in and be passed to different pipelines to perform various operations. In a parallel architecture you can end up with duplicates if the same image is accidentally passed in multiple times especially in the case of extracting from a video feed.
If the identifier in the array of results from the different extending pipelines is a unique_id numeric value we’ll just reindex the values and pass the duplicates along as we’ve seen. Given these are exact duplicates we’d rather the array_merge() function acted as it does with string keys. Let’s take a look at what you can do.
If you want to append the second array to the first while not reindexing and not overwriting the elements in our first array we can use the array union operator instead of array_merge().
The numeric keys in the first array will be preserved, and if a key exists in both of the input arrays then the element value in the first array will be passed. The results from the array union look like this:
The way array_merge() works with numeric keys is not a bug! This functionality is laid out in the PHP documentation.
More Examples Of Using The Array_Merge() Function
Let’s look at some unique and exciting ways we can merge arrays.
Php Combine Arrays With Non Array Types
We can cast values to an array in the parameters of array_merge() to merge arrays.
The output is a resulting array with the first value being indexed at [0].
What is The Difference Between Array_Merge() and Array_Merge_Recursive() in PHP?
The key difference between these two array merging functions is what they do when the arrays have the same keys. Instead of overriding and replacing the keys as array_merge() does, the array_merge_recursive() function makes an array for the element.
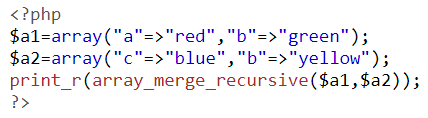
In this example above the element in question is “b”. As we’ve seen before the array_merge() function would remove the duplicate key element in some form based on if it was a string key or numeric key. In the case of array_merge_recursive we’re going to have an element that is an array with “green” and “yellow” as values in the array.
Array ( [a] => red [b] => Array ( [0] => green [1] => yellow ) [c] => blue )
Quick note: Two or more arrays can be merged via the spread operator as well.
Common Error: Array_merge(): Argument #2 Is Not An Array
Let’s look at different situations that lead to this very common error.
One of the Input Arrays is not an Array
As you can imagine this is the most simple explanation for this error. One or more arrays you’re passing in is not an array, and either needs to be adjusted or cast to an array.
Summary
Array_Merge() works to merge arrays together and append array elements to each other. Arrays with matching keys create different logic when matching key’s elements together based on if we have the same string keys or numbered keys.