Php in_array() Function | How To Check If A Value Is In An Array PHP
Let's look at how we can use the PHP in_array() function to check if a value exists in an array.
The PHP in_array() function is a built in PHP function that checks if a given value exists in an array or not. The useful function returns TRUE if the value exists in the given array and FALSE if it does not.
PHP In_Array() Function Syntax

- In this example we are searching for a $needle variable in a $haystack array.
- The $strict variable refers to the type of comparison used between the searched value and the array.
- By default the php in_array() function is using loose comparison unless strict is set to true.
- When $strict is set to true the function will also check and compare the types assigned to the $needle and $haystack.
Few Tips When Checking Value Exists In An Array
- Strict comparison is also known as “===” in PHP.
- As we pointed out above the in_array() function returns true if the value exists in the array, and false if not.
- If the value being compared ($needle) is a string, the in_array() function will use case-sensitivity by default when comparing to values in the array ($haystack).
PHP In_Array() Function Examples

In this example the first if statement passes fine, but the second if statement will fail. Strings are case sensitive and the exact string “Rose” does not exist with a capital “R”.
PHP In_Array() Function Test With Strict Comparison
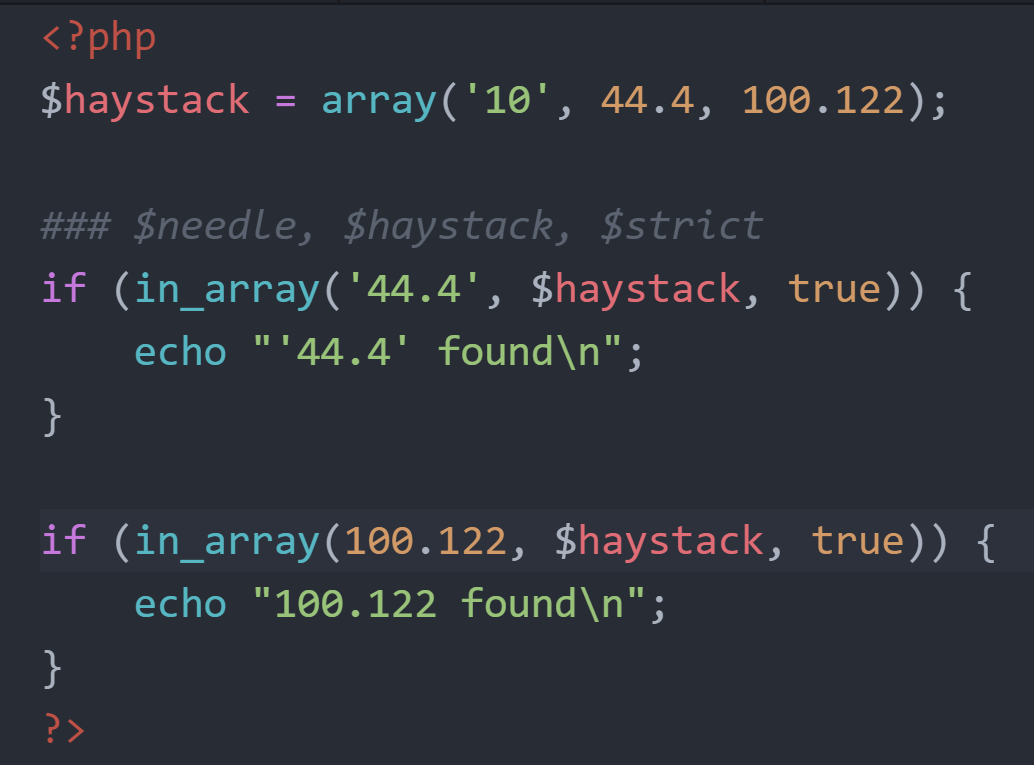
The above example will only pass one of the two tests again. We’ve set $strict check to TRUE which means insensitive type checks are turned off. We do have a value in our $haystack array with 44.4 but our $needle is a string and the array value is not. If you’re running into issues with in_array() not working it's a good idea to check for type mismatches.
PHP In_Array() Function With Array As The Searched Value
We can use an array as the input $needle value and search an array of arrays for matches.
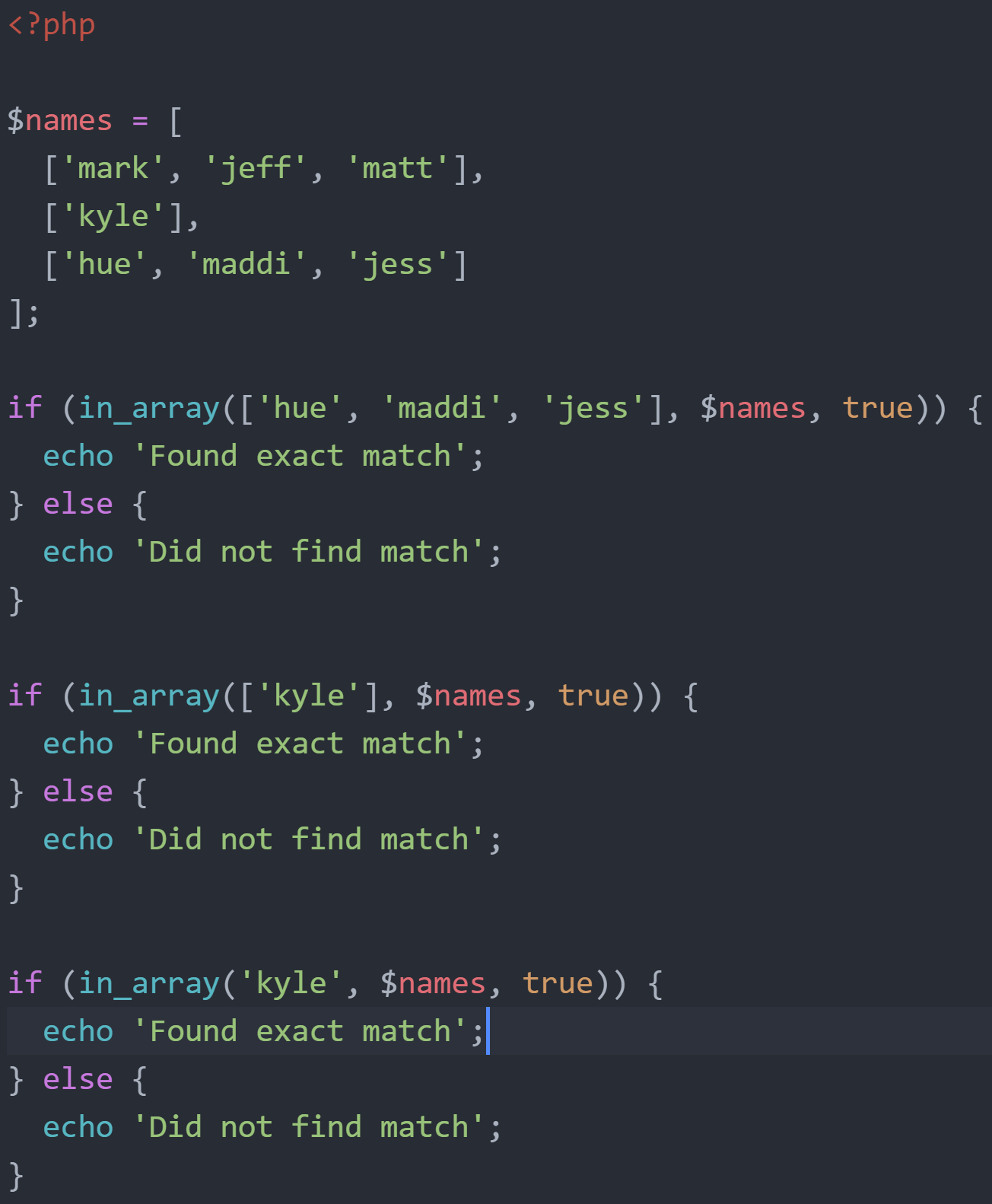
In this example only the first two value searches return True. The last comparison does not have the same types between $needle and $haystack.
Using The PHP In_Array() Function For Multidimensional Arrays To Compare Elements
The in_array() function does not work out of the box for multidimensional arrays when trying to compare the $needle to each element in the multidimensional array. As we saw above we can compare input $needle arrays to each $haystack array as a whole, but not each element.
Our Function For Multidimensional Array Comparison
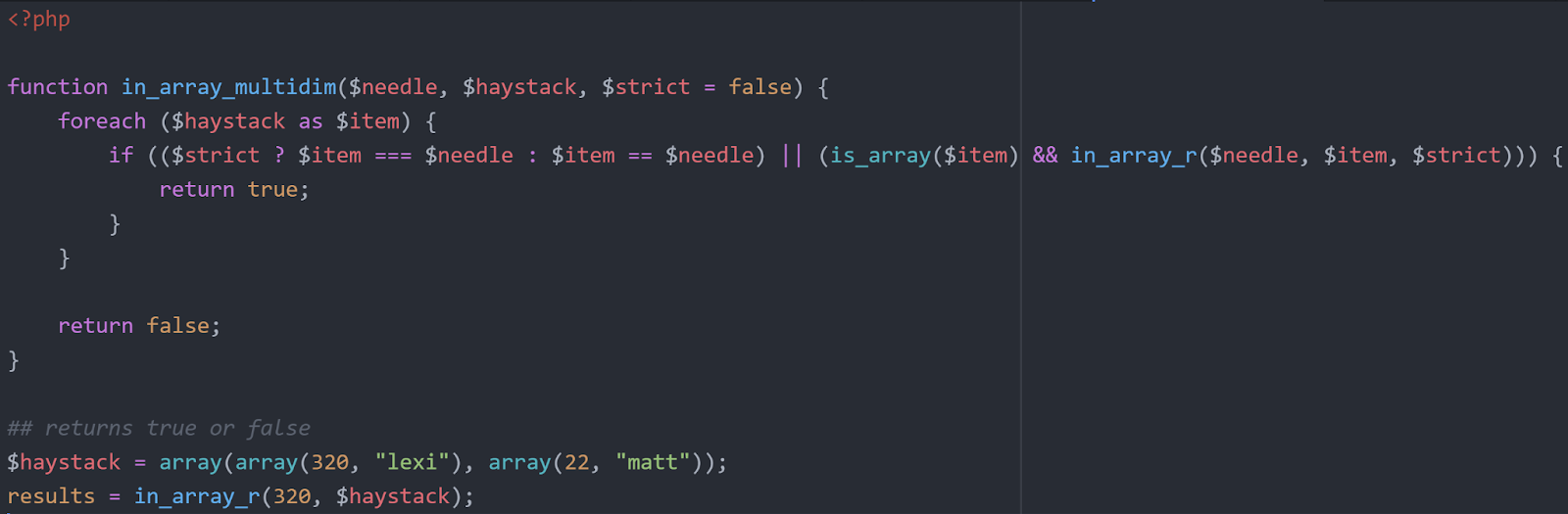
We can create our own input function code for both the $needle value and $haystack and compare each individual element. When the if statement is true then the in_array multidim returns true and false otherwise.
Tips For Troubleshooting When In_Array() Function Is Not Working
One of the most widely seen causes for issues when using the php in_array() function is typing issues when using the default loose comparison. Remember the function will use a loose comparison unless strict is set to true. PHP is extremely lenient on variable types especially in arrays which in real use cases is almost never what we want. Here’s an example:

The last four comparison checks should never come back as true. Remember the php in_array() function is using loose comparison unless $strict is true. By definition of what the function does with loose comparison this is actually correct. We have to define using strict comparison to get the results that make sense given what we were looking for.
In_Array() Function Null Issue
One troubleshooting issue to check if you feel you are missing a key error is if your input array is null and you have strict comparison set to false. When you pass null as the array and use the strict mode set to false your returned result will be null. In this same scenario with strict set to true the in_array() function will throw a TypeError.

Case Insensitive PHP In_Array() Function Use
One of the troubleshooting issues you might run into is realizing that the in_array function is not case insensitive. Strings are compared with exact comparison and there isn’t a parameter in the php function to adjust that. We want to search $haystack for $needle no matter the case of either string. A quick fix for this is writing a simple function to handle case sensitivity for us.

This function checks if a lowercase string value exists in an array by using an array_map when passing the $haystack in. Even when using array_map to handle case insensitivity the in_array() function searches $haystack for $needle using the same process as before.
PHP In_Array() Function To Find a Key In An Array
By default the in_array() function only checks if a value exists in an array compared to the elements of the array. If you want to check the keys in an associative array the best option is actually to use the array_key_exists() function instead. It allows you to pass the same parameters to find the $needle in the $haystack as before.
What's the difference Between PHP In_Array() Function and Array_Search() Function?
The key difference between the in_array() function and the array_search() function in PHP is the in_array() function just checks if the given value exists at all in the array and returns a boolean, whereas array_search() returns the key after searching the array. Confusing these two functions can become time consuming if your array does not have keys and you are moving back and forth between functions.
Runtime Comparison Between In_Array() Function and Array_Search() Function
With smaller array sizes the php in_array function is just slightly worse in total runtime. At an array size of 1000 array_search takes on average 1.346 seconds in total time compared to the 1.41144 seconds for the in_array function.
Interestingly enough as the array sizes grow the in_array function becomes faster in average total runtime. At an array size of 10000 in_array() is a whole .16 seconds faster and that number grows as the size continues past 10000. Both functions are much slower when $strict is set to true because of the stricter type comparison.
Summary
In_Array() is a great way to determine if a given value exists in an array. The function searches $haystack for $needle and returns true or false otherwise. The third parameter $strict lets us adjust if we use strict comparison that includes matching types or loose comparison with much less boundaries.