PHP Str_Replace() Function - How To Replace Characters In A String In PHP
Let’s take a look at how we can use the str_replace() function to replace characters in strings.
Let’s take a look at how we can use the str_replace() function to replace all occurrences of a given string inside another string. We’ll walk through various implementations and examples that show the extension of this php function.
PHP Str_Replace Function Introduction & Syntax
The PHP str_replace() function is a built-in text processing function that is used to replace all the occurrences of a given search string or array with a replacement string or array in a given string or array. The function returns a string or array with all occurrences of our $searchValue replaced with the $replaceValue in our target value.

Parameters For Str_Replace() Function:
The first three parameters can be a string or an array and are mandatory, with the $count parameter being optional.
SearchValue
This is the value either a string or array that we are searching for, sometimes called the $needle. Using an array signals there are multiple strings we want to search for in our target.
ReplaceValue
The replacement value can either be a string or an array and is the value we want to replace with.
SubjectValue
The string or array we want to search and replace on. Sometimes this parameter is referred to as the $haystack. If this parameter is an array then the function performs the search and replace on every element in the subjectValue and the return becomes an array.
Count
An optional fourth parameter that can be used to set the number of replacements we want to remove. The count parameter was added in PHP 5.0.
Return Values For Str_Replace() Function:
The function returns either a string or an array with the replacement values. If arrays were used for subjectValue then we return an array of each element the function performed a search and replace on.

If we pass arrays for both our $searchValue and $replaceValue parameters, the php str_replace() function uses each value in the $searchValue array and replaces it with each string in the $replaceValue array like a double for loop.
In the case where the $replaceValue array contains less elements than the array used for $searchValue parameter the str_replace() function uses an empty string for the replacement.
In the case where the $searchValue parameter is an array but the $replaceValue parameter is a string the str_replace() function replaces each element in the array with the $replaceValue string.
The str_replace() function does not affect the original $subjectValue string but returns a new copy of the $subjectValue string. This function is perfect when you don’t need complex regular expression type replacements.
Quick Notes About The PHP Str_Replace() Function:
This function replaces values from left to right in the string which means in the case of multiple replacements (with an array) the function might replace newly inserted values with later values from the $searchValue array.
This function is designed to be case-sensitive and you should use str_ireplace for case-insentive replacement.
PHP Str_Replace() Function Examples:
Let’s look at a wide variety of ways to use the str_replace() function.
Simple PHP String Replace Examples:
This code example performs a string replace using the str_replace function with all strings as input. We replace the string ‘PHP’ with ‘coding’ in the target string $string1
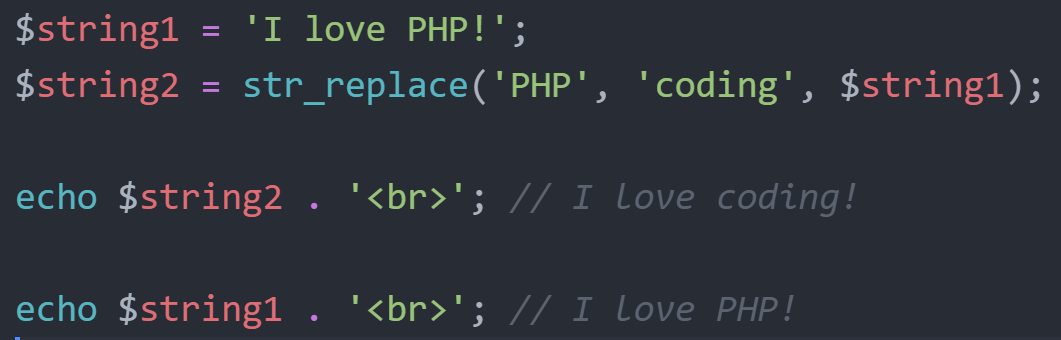
You’ll notice when running this our $string1 is exactly the same as it was before performing a string replace on our $subjectValue. Str_replace does not change the original string but recreates a new one and returns it to $string2.
The following code snippet shows that we can replace multiple instances of the same string with a new $replaceValue string.
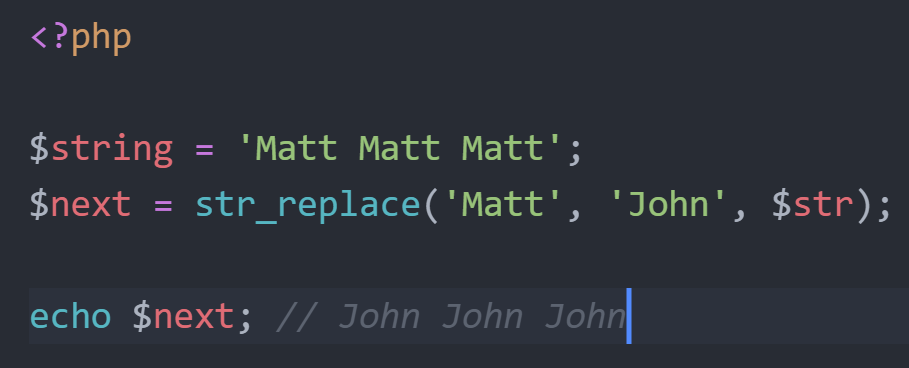
Str_Replace Function With Multiple Replacements & Searches
This example below uses str_replace() function to replace any instance of the words ‘Monday’, ‘Tuesday’ or ‘Wednesday’ with different days ‘Friday’, ‘Saturday’, and ‘Sunday’ in the original string "Today is Monday, Tuesday, or Wednesday".
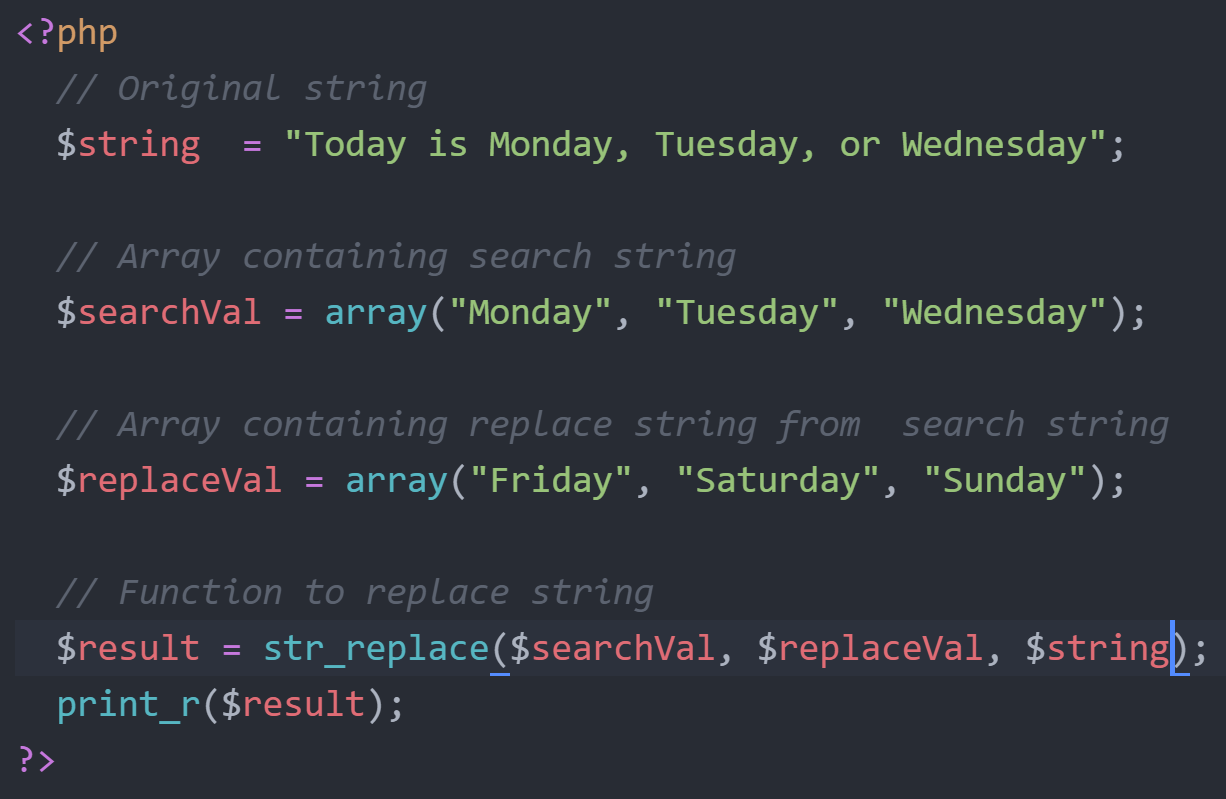
Like we pointed out above, the str_replace() function replaces instances from left to right and can lead to replacing instances we’ve already replaced. Words that are replaced and added to our $subjectValue string that contain another replacement word can fall victim to this.
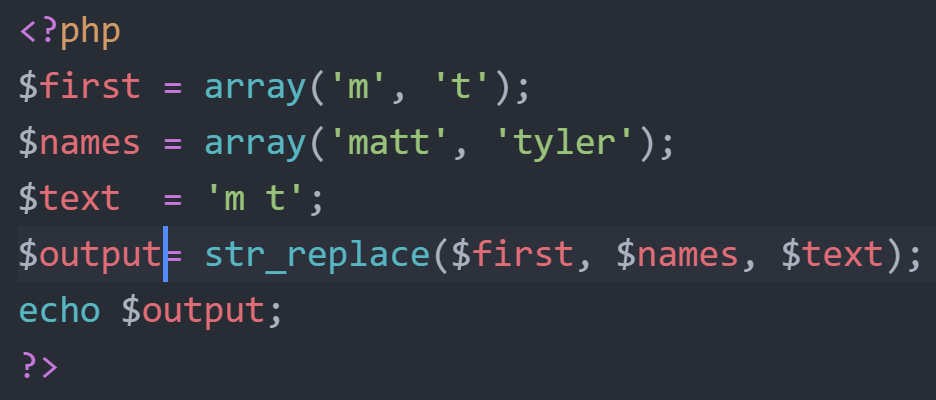
In this example the ‘m’ will be replaced with ‘matt’ and then each ‘t’ becomes a ‘tyler’ string. We end up with three different instances of ‘tyler’.
Tips From A Real Str_Replace() Use Case
While building this product matching software we used web scraping to grab data we could use as training data for the different models we used. This data was raw and contained many characters and symbols that needed to be removed. The str_replace() function is sometimes used to cleanse and parse important data from web scraped data or text file data. There are a few things you want to keep in mind in a use case like this.
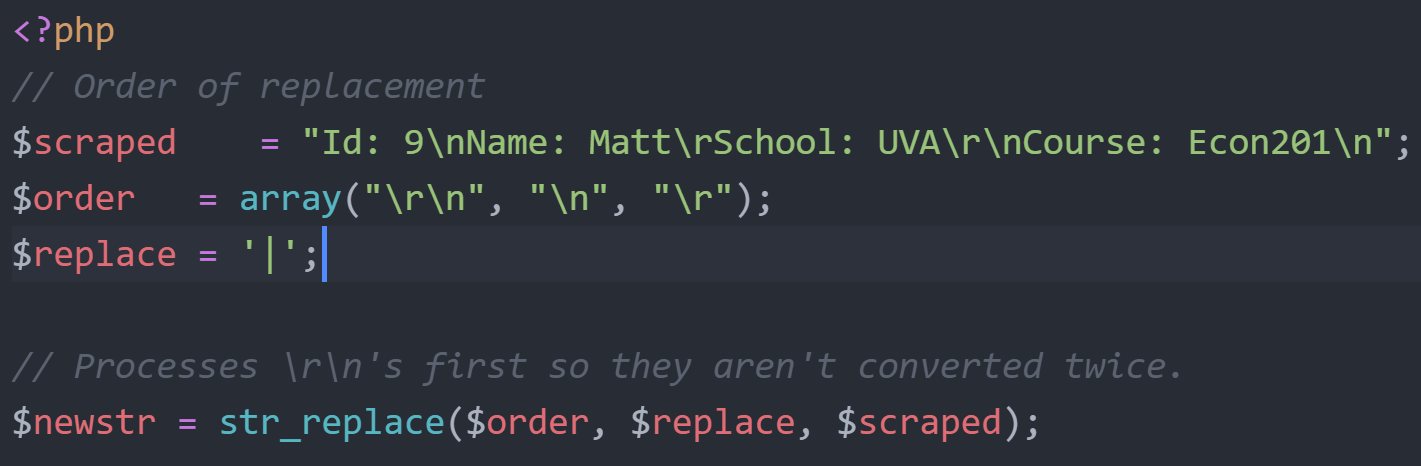
When replacing patterns containing similar characteristics, always list the most robust patterns first. Many of the patterns you’ll find will be similar to each other or just a concatenation of two sub patterns. Watch for this as you plan your search array.
Try to make sure as best you can that your new replacement patterns don’t affect the data you want to extract. Removing entire sections of an input string can always lead to changes to how the data you care about reads.
Deeper Examples Of PHP String Replacement Using Str_Replace()
Let’s look at some powerful and efficient ways to use the str_replace() function to perform different tasks.
Faster String Replacement With Multiple $SubjectValue Strings
When running an array of $subjectValue strings through the same searches and replacements it's almost 3x faster to use json_encode() on the $subjectValue array, use the str_replace() function, then json_decode() at the end. The function returns our new string with replaced values.

Note: You might want to set a second parameter to ‘True’ in the json_decode function as this will return a type object by default instead of the expected array. Be careful with what your $searchValue and $replaceValue are as well. If they contain strings that match json encoding it can lead to issues.
Replace Just The First Occurrence Of A Search Value
This example function replaces only the first occurrence of the search string in the $subjectValue string. Although this does not use str_replace() this method has the fastest runtime of all the available options. Example taken from this stackoverflow post.

In the above code $haystack is our subject string we want to search and replace. $Needle is the search string and $replace is the replacement string.
Convert A String Into a URL With PHP Str_Replace & Others
Let’s say we want to convert a string such as “This is totally, my URL?” into a URL slug used for a website. We can write a function that uses preg_replace(), strtolower(), and str_replace PHP functions.

The original string from above now becomes “this-is-totally-my-url” after passing through this function. We use strtolower() to convert this string to all lowercase, then preg_replace let’s us use regular expressions to make replacements in our string, then finally we use str_replace() to add ‘-’. Turned at the end is a replacement string with any outstanding ‘-’ characters trimmed.
Quick Note: preg_replace() replaces found search values with a replacement string just like we can with str_replace(). We can also designate multiple replacements with an array.
Can I Use The PHP Str_Replace() Function For Associative Arrays?
An associative array with keys as the search values and array values as the replacement strings can be broken down and passed into str_replace() by grabbing the array_keys and array_values separately from the associative array. The following example assumes you’re using a string for the $subjectValue to search.

Multiple replacements now becomes a little bit cleaner as we can no longer have an instance where the number of replacement values and search values will be the same, assuming no nulls.
Summary
Str_Replace() is a great case sensitive way to search a given string or array of strings for strings and replace them without fancy replacing rules. The function allows you to designate multiple needles ($searchValue), multiple replacement strings, and multiple $subjectValue strings. On top of this the function replaces found search values quickly with a faster runtime than functions like preg_replace.